Catch2 Tutorial: Getting Started with C++ Unit Testing
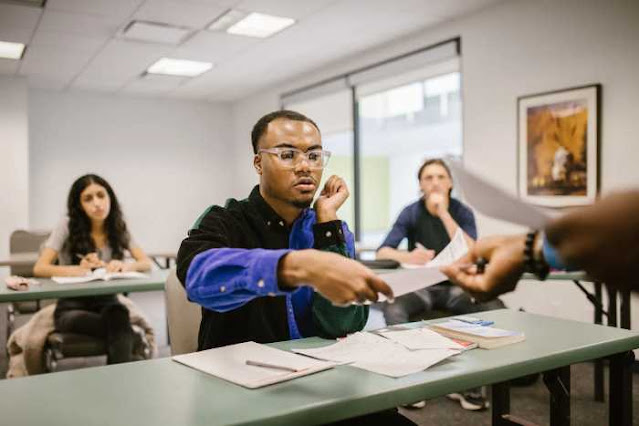
Introduction
Unit testing is a critical practice in software development
that ensures individual units of code work as expected. It helps catch bugs
early, improves code quality, and boosts developer confidence. Catch2 is a
popular C++ unit testing framework known for its simplicity and ease of use. This
tutorial will guide you through getting started with Catch2 for C++ unit
testing.
Installing Catch2
Before we begin, you need to install Catch2 on your system.
Catch2 is a header-only library, which means you only need to download the
header file to use it. You can get the latest version of Catch2 from its GitHub
repository:
Once downloaded, add the Catch2 header file to your C++
project's included path.
Writing Your First Test
Let's start by writing a simple test using Catch2. Create a
new C++ source file and include the Catch2 header at the top:
cpp
Copy code
#include <catch2/catch.hpp>
Next, define a test case using the TEST_CASE macro:
cpp
Copy code
TEST_CASE("Addition Test", "[math]") {
int result = 2 +
2;
REQUIRE(result ==
4);
}
In this example, we created an "Addition Test"
test case belonging to the "math" test suite. The test case checks
whether adding 2 and 2 equals 4 using the REQUIRE macro. If the state is false,
the test fails.
Compiling and Running Tests
To compile your test suite, you can use any C++ compiler
that supports C++11 or later. For example, using G++:
c
Copy code
g++ -std=c++11 your_test_file.cpp -o tests
Now, run the compiled executable:
bash
Copy code
./tests
You should see the output indicating the number of test
cases, test suites, and whether all the tests passed.
Writing More Tests
Catch2 allows you to write and group multiple test cases
into test suites. Test suites help organize related tests. Here's an example of
numerous test cases in different test suites:
cpp
Copy code
#include <catch2/catch.hpp>
TEST_CASE("Addition Test", "[math]") {
int result = 2 +
2;
REQUIRE(result ==
4);
}
TEST_CASE("Subtraction Test", "[math]")
{
int result = 5 -
3;
REQUIRE(result ==
2);
}
TEST_CASE("String Test", "[utilities]")
{
std::string str =
"Catch2";
REQUIRE(str.length() == 6);
}
In this example, we have three test cases: "Addition
Test" and "Subtraction Test" are part of the "math"
test suite, while "String Test" belongs to the "utilities"
test suite.
Using Sections
Catch2 provides a " Sections " feature that allows
you to break down a test case into smaller, more manageable parts. Sections are
helpful when you want to test different scenarios within the same test case:
cpp
Copy code
TEST_CASE("Multiplication Test",
"[math]") {
int a = 3;
int b = 4;
SECTION("Positive Numbers") {
int result = a
* b;
REQUIRE(result
== 12);
}
SECTION("Zero
and a Positive Number") {
int result = 0
* b;
REQUIRE(result
== 0);
}
SECTION("Negative Numbers") {
int result =
(-a) * (-b);
REQUIRE(result
== 12);
}
}
In this example, we have a test case named
"Multiplication Test" with three sections that test multiplication
under different scenarios.
Using Matchers
Catch2 provides various matches to check conditions in
tests. Matchers make your test cases more expressive and readable:
cpp
Copy code
TEST_CASE("Matchers Test",
"[utilities]") {
std::vector<int> numbers = {1, 2, 3, 4, 5};
REQUIRE_THAT(numbers, Catch::Equals(std::vector<int>{1, 2, 3, 4,
5}));
REQUIRE_THAT(numbers, Catch::Contains(3));
REQUIRE_THAT(numbers, Catch::AllOf(Catch::VectorContains(4),
Catch::VectorContains(5)));
}
In this example, we use various matches to test the numbers
vector.
Conclusion
Unit testing is a crucial aspect of modern software development,
and Catch2 provides an excellent framework for C++ developers to write
efficient and expressive test cases. This tutorial covered the basics of
getting started with Catch2, including installation, writing simple test cases,
organizing them into test suites, using sections to test different scenarios,
and leveraging matches to enhance test readability.
With Catch2, you can confidently write robust C++ code while
ensuring that your application's individual components function as expected. Incorporating
unit testing into your development workflow allows you to catch bugs early,
reduce maintenance costs, and deliver high-quality software to your users.
Happy testing!
Comments
Post a Comment